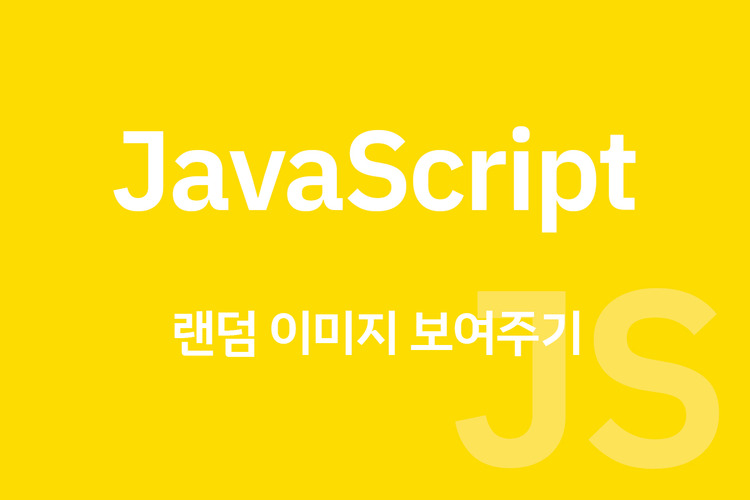
[JavaScript] 랜덤 이미지 보여주기
Lpla
·2021. 2. 18. 21:05
반응형
자바스크립트로 랜덤 이미지를 보여주는 방법에 대해 알아보겠다.
0. 기본값 세팅
HTML
<body>
<div class="container">
<div class="img_box"></div>
</div>
<!-- jQuery cdn -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.5.1/jquery.min.js" integrity="sha512-bLT0Qm9VnAYZDflyKcBaQ2gg0hSYNQrJ8RilYldYQ1FxQYoCLtUjuuRuZo+fjqhx/qtq/1itJ0C2ejDxltZVFg==" crossorigin="anonymous"></script>
</body>
CSS
html, body { width: 100%; height: 100%; margin: 0; }
.container { position: relative; width: 100%; height: 100%; }
.img_box { width: 100%; height: 100%; position: relative; left: 50%; transform: translateX(-50%); background-repeat: no-repeat; background-size: cover; }
먼저 HTML에는 container와 img_box 클래스로 된 요소 2개를 만들고 하단에 제이쿼리를 불러온다.
그리고 CSS는 각자 맞는 스타일을 지정하면 된다.
1. background-image
CSS
.img_box.bg01 { background-image: url(/images/bg01.jpg); }
.img_box.bg02 { background-image: url(/images/bg02.jpg); }
.img_box.bg03 { background-image: url(/images/bg03.jpg); }
.img_box.bg04 { background-image: url(/images/bg04.jpg); }
CSS를 이렇게 세팅하더라도 아직 초기 화면에는 아무것도 보이지 않는다.
이제 자바스크립트로 img_box에 클래스를 추가하여 이미지를 보여줄 수 있다.
자바스크립트로 특정 요소에 클래스를 추가하는 방법은 아래와 같다.
바닐라 자바스크립트
let randomNumber = Math.floor(Math.random() * 4) + 1;
document.querySelector('.img_box').classList.add('bg0' + randomNumber);
제이쿼리
let randomNumber = Math.floor(Math.random() * 4) + 1;
$('.img_box').addClass('bg0' + randomNumber);
2. img태그
상황에 따라 background-image를 사용하지 않고 img태그로 랜덤 이미지를 구현하고 싶을 수 있다.
HTML
<div class="container">
<div class="img_box">
<img src="/images/bg01.jpg" alt="배경이미지">
</div>
</div>
CSS
img { width: 100%; height: 100%; }
먼저 이미지 태그를 미리 만들어둔다.
하지만 이미지 주소를 처음부터 비워둘 필요는 없고 아무 이미지로 경로를 채우는 것이 좋다.
바닐라 자바스크립트
let randomNumber = Math.floor(Math.random() * 4) + 1;
document.querySelector('.img_box img').setAttribute('src', '/images/bg0' + randomNumber + '.jpg');
제이쿼리
let randomNumber = Math.floor(Math.random() * 4) + 1;
$('.img_box').children('img').attr('src', '/images/bg0' + randomNumber + '.jpg');
이 방식은 이미지 파일명이 bg01.jpg, bg02.jpg .... 일 경우만 사용 가능하다.
하지만 현실적으로 그렇지 않은 파일명이 더 많을 것이다.
이때는 배열을 이용하면 된다.
배열을 이용하는 방법
let randomNumber = Math.floor(Math.random() * 4);
let imgName = ['forest', 'lake', 'nature', 'winter'];
$('.img_box').children('img').attr('src', '/images/' + imgName[randomNumber] + '.jpg');
배열의 1번값은 [0]이기 때문에 Math.random() * 4 + 1
이 아닌 Math.random() * 4
를 사용해야 한다.
반응형